The big part of Rust developers is focused on Linux systems. As a windows developer you don’t want to install a VM or even another linux partition on your system. This is where WSL2 comes into play. In this short introduction i will demonstrate how you can setup your windows system to be able to develop linux applications.
As an example I will use the substrate node template, which is an blockchain project and the reason I even looked into this topic. After proper compilation this error was the only thing I could get out of my Substrate node:
thread 'main' has overflowed its stack
Nice. I figured out that the stack size differs between the operating systems. I could try to fix it just to find another issue afterwards or switch to linux. And thats what we are doing now.
Prepare your windows system
First you need to make sure you have intalled WSL 2, which can easily be done by following this detailed instruction. Afterwards install a Linux distribution like ubuntu from the windows store.
Afterwards you should install Visual Studio Code as the development IDE. It can be found here. Make sure to install the windows version, as we still want to develop on our windows machine.
After you started VSCode you need to install the WSL extension. Now you can select WSL as file system with the lower left button.
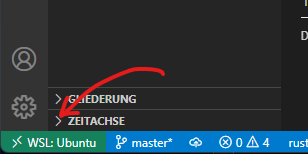
You also need to install CodeLLDB as a debugger and a rust plugin like rust-analyzer. You can also install a Terminal for convenience. Make sure those are installed within the Ubuntu wsl.
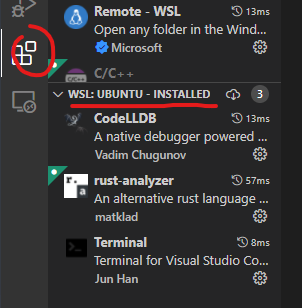
Prepare your linux system
Now open a terminal in VSCode or just by opening the Linux distribution.
By default the whole windows PATH is imported into the linux terminal. This is unecessary and can cause trouble if programms are installed seperately on windows and linux. To disable this behavior you need to modify the file wsl.config file as root. It could be that the file doesnt exists. In this case create one.
sudo nano /etc/wsl.conf
From windows this file can be found at within the explorer.
\\wsl.localhost\Ubuntu\etc\wsl.conf
Add the following lines
[interop] appendWindowsPath = false
and restart your wsl by executing
wsl --shutdown
as Admin within a windows Terminal.
Install Rust
Install requierd packages first.
sudo apt update && sudo apt install -y git clang curl libssl-dev llvm libudev-dev
Now we can install rust. This is easeally done with the rustup script. Just type the following command into your linux terminal and your are done.
curl https://sh.rustup.rs -sSf | sh
This will install rustup and cargo as well as the rust compiler. Afterwards we should add cargo to our PATH environment variable
source ~/.cargo/env
and update rust.
rustup update
You can check the installation with a version call to the rust compiler. And
rustc --version
Build and debug your application
As already mentioned we use the substrate node template as an example. Clone the git repository and cd into it.
git clone https://github.com/substrate-developer-hub/substrate-node-template && cd substrate-node-template
Now you can build the application. This will propably take a few minutes.
cargo build
You can start a debug session by clicking on the Run menu in VSCode and select Run or simply use F5. If you do this the first time you wan’t have a run configuration. VSCode will install one automatically. If not copy the following into the path ./.vscode/launch.json.
"version": "0.2.0", "configurations": [ { "type": "lldb", "request": "launch", "name": "Debug executable 'node-template'", "cargo": { "args": [ "build", "--bin=node-template", "--package=node-template", ], "filter": { "name": "node-template", "kind": "bin" } }, "args": ["--dev", "--tmp"], "cwd": "${workspaceFolder}" }, { "type": "lldb", "request": "launch", "name": "Debug unit tests in executable 'node-template'", "cargo": { "args": [ "test", "--no-run", "--bin=node-template", "--package=node-template" ], "filter": { "name": "node-template", "kind": "bin" } }, "args": [], "cwd": "${workspaceFolder}" } ] }
This does nothing more than telling VSCode how to build with cargo and that we use lldb as debugger.
Thats it. You should now be able to develop rust applications within WSL 2 as linux environment.